Writing to the PHP error_log with var_dump & print_r
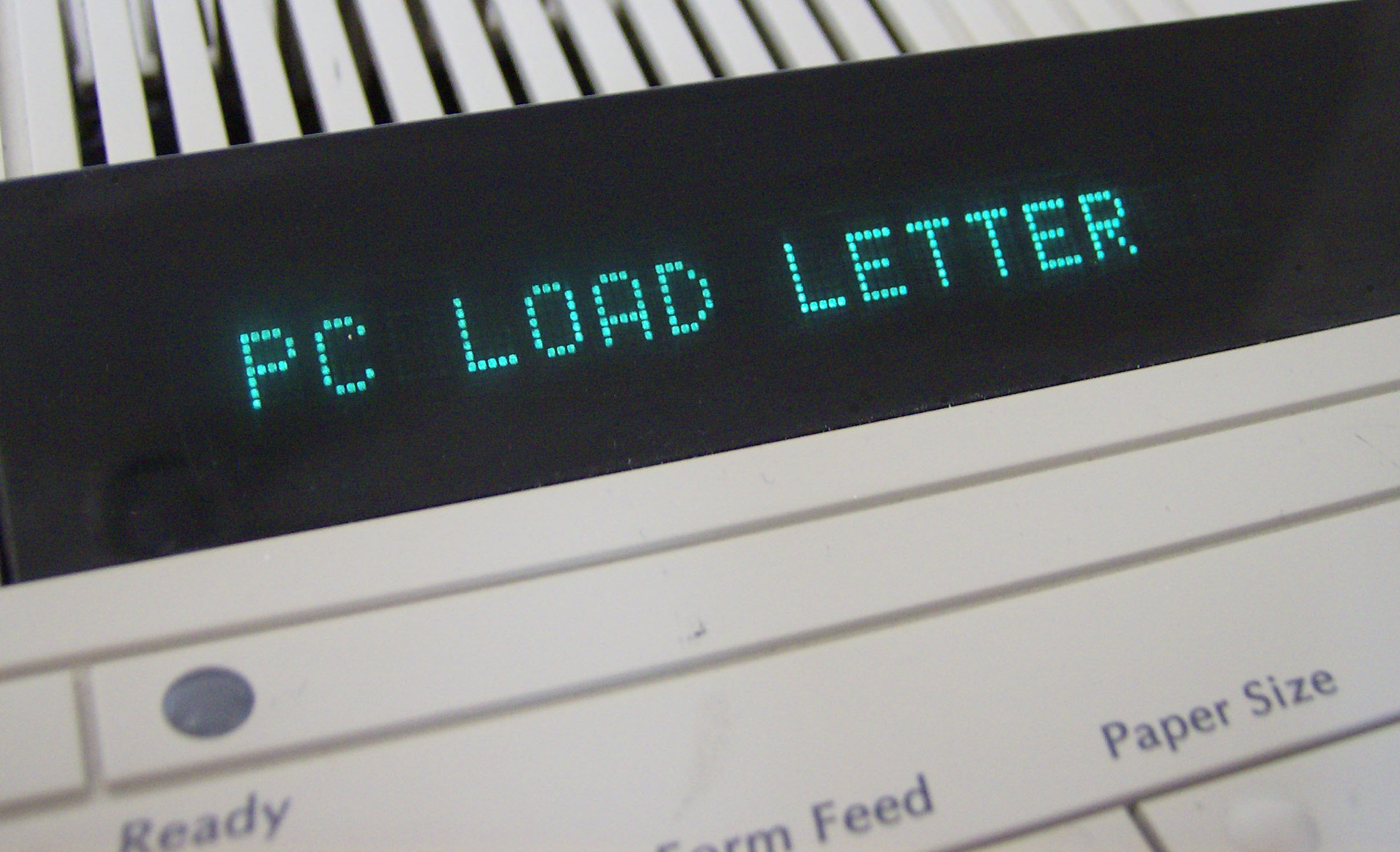
Writing objects to the PHP error log
using error_log()
can be useful, but it isn’t allowed – you can only write strings there. What to do?
Well, luckily there are options for both print_r()
– human friendly – and var_dump()
– parseable – that will let you convert an object
into a string
.
Use print_r()
Using print_r()
is the easiest, since the second parameter to the function says that we want to send the output to a variable rather than echoing it. Thus, to write to the error_log
you just need to use the following code.
$object = new MyObject(); error_log( print_r( $object, true ) );
Use var_dump()
If you want the more detailed output of var_dump()
, it’s a bit trickier, but still pretty easy. In a nutshell, since you can only echo the results you have to capture the output buffer with ob_start()
, assign it to a variable, and then clean the buffer with ob_end_clean()
allowing you to write the resulting variable to the error_log
.
function var_error_log( $object=null ){ ob_start(); // start buffer capture var_dump( $object ); // dump the values $contents = ob_get_contents(); // put the buffer into a variable ob_end_clean(); // end capture error_log( $contents ); // log contents of the result of var_dump( $object ) } $object = new MyObject(); var_error_log( $object );
Thanks so much. Wanted to output the response headers for the a WordPress function (wp_remote_get). Can’t find better way than this.
great stuff. this is a major help.
Nice write-up! Perhaps another option for your readers: When I need the OOP-related detail of var_dump, then I tend to use:
error_log(var_export($message, true));
This is great stuff, thank you very much for sharing!
thanks for sharing your knowledge! cheers
Nice! Saved on my favorites! thanks!
This is a question that was brought up in my Programing Fundamentals class
when should you use a var_dump and when should you use a print_r
could someone help me understand it.
Hi Robert – this is a pretty good explanation of the differences: http://stackoverflow.com/questions/3406171/php-var-dump-vs-print-r
Hello! I’ve been following your blog for a long time
now and finally got the courage to go ahead and give you a shout
out from Atascocita Texas! Just wanted to mention keep up the good job!
Hey man,
very cool and simple trick, helped me a lot recently 🙂
Very cool, man. To be honest, I’m having trouble finding the error_log. Is it supposed to create a folder and/or file in wp-content when debug is set to true? No – there it is – it just writes it to the php error log as set in php.ini.
Anything you send to error_log() ends up in the PHP error log, but the location of that depends on how you have your server configured. It is usually something like /var/log/httpd/error.log for Apache, /var/log/nginx/error.log for Nginx. If you are using Windows or OSX it will depend on your config.