Salesforce sObject Id Validation in Apex
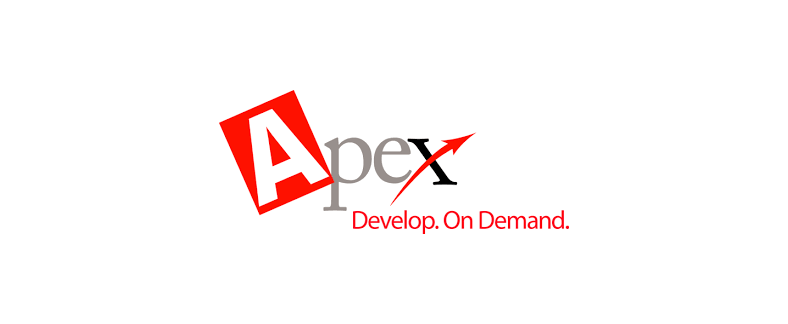
Salesforce sObject Id‘s are specially formatted strings used to uniquely identify sObjects. If you want to test the validity of an sObject Id in Apex, you can go the simple route of checking the length, the slightly more complex route of writing a regular expression, or even go as far as to implement the logic parsing of ID values. An easier way to get accurate testing of validity is to create an instance of the sObject you want to test against, then try to assign your sObject Id to it, and catch any Exceptions. This will validate both the format, as well as the type of the sObject Id.
The Util Code
public abstract class MyUtilClass { /** * Test a String to see if it is a valid SFDC ID * @param sfdcId The ID to test. * @param t The Type of the sObject to compare against * @return Returns true if the ID is valid, false if it is not. */ public static Boolean isValidSalesforceId( String sfdcId, System.Type t ){ try { if ( Pattern.compile( '[a-zA-Z0-9]{15}|[a-zA-Z0-9]{18}' ).matcher( sfdcId ).matches() ){ // Try to assign it to an Id before checking the type Id id = theId; // Use the Type to construct an instance of this sObject sObject sObj = (sObject) t.newInstance(); // Set the ID of the new object to the value to test sObj.Id = id; // If the tests passed, it's valid return true; } } catch ( Exception e ){ // StringException, TypeException } // ID is not valid return false; } }
You can then test the validity of the sObject Id by passing it into the util method along with the System.Type of the sObject you want to compare against. The type can be accessed using sObject.class
.
Call From Apex
// maybe a valid Account.Id, maybe not? String theId = '001xxxxxxxxxxxx'; if ( MyUtilClass.isValidSalesforceID( theId, Account.class ) ){ // do something if it is valid }
Excellent solution! It worked for me.
Hi,
How about using instanceof keyword to check this String value is an Id. Then check the sObject Type matches what you are looking for.
That’s a good solution – have you tested it? Coming from a Java background it doesn’t immediately make sense to me that
String instanceof Id == true
, but in the Apex world I don’t see a reason why it wouldn’t, and I would think it’s more efficient.J